Pointers with 1-D arrays in programming allow efficient memory management and data access. A pointer can store the base address of a 1-D array, enabling traversal and manipulation of array elements using pointer arithmetic. This powerful combination simplifies operations like element access and iteration, making code more flexible and efficient.
Lets take an example 1-D array
int arr[10] = {4, 2, 6, 4, 1, 8, 4, 6, 1, 8};
The variable arr
is an array, and it represents the address of the first element, which is equivalent to &arr[0]
. As explained in the pointers chapter, when an address is dereferenced, the value stored at that address is obtained. To access the value of the first element of the array, *arr
can be used.
To access subsequent elements, the expression *(arr + 1)
retrieves the second element, *(arr + 2)
retrieves the third, *(arr + 3)
retrieves the fourth, and so on. This method accesses elements by calculating their addresses and then dereferencing those addresses to obtain their values, rather than using the typical index notation like arr[0]
, arr[1]
, arr[2]
, etc.
Lets take a sample program:
#include <stdio.h>
int main()
{
int arr[5] = {4, 2, 6, 4, 1};
int i = 0;
for (i = 0; i < 5; i++)
printf("address at %d = %p, value = %d\n", i, arr + i, *(arr + i));
}
output:
address at 0 = 0x7fffffffdc90, value = 4
address at 1 = 0x7fffffffdc94, value = 2
address at 2 = 0x7fffffffdc98, value = 6
address at 3 = 0x7fffffffdc9c, value = 4
address at 4 = 0x7fffffffdca0, value = 1
Pointer holding address of an array
As we know, Pointers hold the address of a variable. In this context, arr
is an array variable that inherently holds the address of its first element. When a pointer is assigned the base address of the array, it points to this base address. Using that pointer, it becomes easy to traverse through the entire array.
An array can be assigned to pointer as
int arr[6] = {14, 65, 23, 89, 34};
int *ptr = arr;
or
int arr[6] = {14, 65, 23, 89, 34};
int *ptr = &arr;
or
int arr[6] = {14, 65, 23, 89, 34};
int *ptr = &arr[0];
All of the above initialisations are same and pointer holds the base address of array.
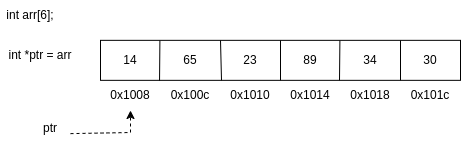
When a pointer holds the base address of an array, it gains access to the entire array, allowing both reading and writing data to any index value within the array. Let’s consider a pointer that points to an array of integers, ideally pointing to the first index. By dereferencing the pointer, we retrieve the value at the first index. If we wish to access a specific index position, we can do so using multiple methods as below
1. ptr[i]
#include <stdio.h>
int main()
{
int i;
int arr[6] = {14, 65, 23, 89, 34, 30};
int *ptr = arr;
for (i = 0; i < 5; i++)
{
printf("arr[%d] is %d, ", i, arr[i]);
printf("ptr[%d] is %d, ", i, ptr[i]);
printf("addr of arr[%d] is :%p", i, &arr[i]);
printf("addr of ptr is :%p\n", ptr);
}
}
output:
arr[0] is 14, ptr[0] is 14, addr of arr[0] is :0x7ffd5110c740, addr of ptr is :0x7ffd5110c740
arr[1] is 65, ptr[1] is 65, addr of arr[1] is :0x7ffd5110c744, addr of ptr is :0x7ffd5110c740
arr[2] is 23, ptr[2] is 23, addr of arr[2] is :0x7ffd5110c748, addr of ptr is :0x7ffd5110c740
arr[3] is 89, ptr[3] is 89, addr of arr[3] is :0x7ffd5110c74c, addr of ptr is :0x7ffd5110c740
arr[4] is 34, ptr[4] is 34, addr of arr[4] is :0x7ffd5110c750, addr of ptr is :0x7ffd5110c740
2. *(ptr + i)
#include <stdio.h>
int main()
{
int i;
int arr[6] = {14, 65, 23, 89, 34, 30};
int *ptr = arr;
for (i = 0; i < 5; i++)
{
printf("arr[%d] is %d, ", i, arr[i]);
printf("ptr[%d] is %d, ", i, *(ptr + i));
printf("addr of arr[%d] is :%p", i, &arr[i]);
printf("addr of ptr is :%p\n", ptr);
}
}
output:
arr[0] is 14, ptr[0] is 14, addr of arr[0] is :0x7ffe83ede680, addr of ptr is :0x7ffe83ede680
arr[1] is 65, ptr[1] is 65, addr of arr[1] is :0x7ffe83ede684, addr of ptr is :0x7ffe83ede680
arr[2] is 23, ptr[2] is 23, addr of arr[2] is :0x7ffe83ede688, addr of ptr is :0x7ffe83ede680
arr[3] is 89, ptr[3] is 89, addr of arr[3] is :0x7ffe83ede68c, addr of ptr is :0x7ffe83ede680
arr[4] is 34, ptr[4] is 34, addr of arr[4] is :0x7ffe83ede690, addr of ptr is :0x7ffe83ede680
3. *ptr and ptr++
In the two cases mentioned the address location to which ptr is pointing will not effect. there is another way of accessing elements in an array but this effects the value in ptr.
#include <stdio.h>
int main()
{
int i;
int arr[6] = {14, 65, 23, 89, 34, 30};
int *ptr = arr;
for (i = 0; i < 5; i++)
{
printf("arr[%d] is %d, ", i, arr[i]);
printf("ptr[%d] is %d, ", i, *ptr);
printf("addr of arr[%d] is :%p ", i, &arr[i]);
printf("addr of ptr is :%p\n", ptr);
ptr++;
}
}
output:
arr[0] is 14, ptr[0] is 14, addr of arr[0] is :0x7fffa46a6ea0 addr of ptr is :0x7fffa46a6ea0
arr[1] is 65, ptr[1] is 65, addr of arr[1] is :0x7fffa46a6ea4 addr of ptr is :0x7fffa46a6ea4
arr[2] is 23, ptr[2] is 23, addr of arr[2] is :0x7fffa46a6ea8 addr of ptr is :0x7fffa46a6ea8
arr[3] is 89, ptr[3] is 89, addr of arr[3] is :0x7fffa46a6eac addr of ptr is :0x7fffa46a6eac
arr[4] is 34, ptr[4] is 34, addr of arr[4] is :0x7fffa46a6eb0 addr of ptr is :0x7fffa46a6eb0
Below program uses pointers to add all elements in an array with value 2
#include <stdio.h>
int main()
{
int arr[10] = {10, 20 ,30 , 40 , 50, 60, 70, 80, 90, 100};
int *ptr = arr;
int size = sizeof(arr)/sizeof(arr[0]);
int i;
while ((ptr - arr) < size) {
*ptr += 2;
ptr++;
}
printf("the array elements after adding with 2:\n");
for(i = 0; i < size; i++)
printf("%d ", arr[i]);
printf("\n");
}
output:
size = 10
the array elements after adding with 2:
12 22 32 42 52 62 72 82 92 102
Prev << Arrays in C
Next >> strings in C