In programming, you often need to perform a similar task repeatedly. But copying the same task over and over makes the program large and difficult to read. That’s when loops in C come into play. Loops allow you to repeat that task many times without making it messy. They help keep your program neat and tidy.
for example if you want to print “hello! onesandzeroverse.com” for 5 times, here is how we can write without loops.
#include <stdio.h>
int main()
{
printf("hello! onesandzeroverse.com\n");
printf("hello! onesandzeroverse.com\n");
printf("hello! onesandzeroverse.com\n");
printf("hello! onesandzeroverse.com\n");
printf("hello! onesandzeroverse.com\n");
}
output:
hello! onesandzeroverse.com
hello! onesandzeroverse.com
hello! onesandzeroverse.com
hello! onesandzeroverse.com
hello! onesandzeroverse.com
As seen in the above program output “hello! onesandzeroverse” is printed for 5 times, but how about getting the same output by using loops.
#include <stdio.h>
int main()
{
int i;
for (i = 0; i < 10; i++)
{
printf("hello! onesandzeroverse.com\n");
}
}
output:
hello! onesandzeroverse.com
hello! onesandzeroverse.com
hello! onesandzeroverse.com
hello! onesandzeroverse.com
hello! onesandzeroverse.com
you can see the difference how the program became compact if we use loops.
C employees various types of loops for, while, do while. each one of them have their own use cases. Wether it is any kind of loop it works on 3 main factors
- a variable assigned with a value.
- condition check for the variable (stays in loop untill this condition failes).
- updation of variable value.
lets see how loops are designed based on these 3 factors
for loop
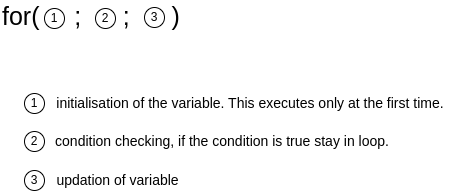
syntax:
for (variable initialisation; condition; update value)
{
/* statements to execute in loop */
}
here is the sample program:
// Write a program which prints first 5 multiples of 5
#include <stdio.h>
int main()
{
int i;
for (i = 1; i <= 5; i++)
printf("%d\n", i * 5);
}
output:
5
10
15
20
25
in the above program i = 1 is the initialisation of the variable, i <=5 is the condition check, i++ is the value updation as we have to print 5 * i, i value have to start from 1 and stop at 5 so we initialised i with 1 and condition is checked as 1 <= 5. The flow the program at loop will be as follows:
- initialise i = 1.
- checks condition i < = 5. condition is true so it excecutes the statement inside loop block
- after executing the statement i is updated i.e i++ which which value of i is incremented by 1, now i = 2.
- checks condition again, 2 <= 5 condition true, step 3 is repeated again.
- step 2 and 3 are repeated untill the condition reaches 6 <= 5. condition is failed, so program counter comes out of loop.
while loop
In case of “for” loop all the 3 factors are in a single line, where are in while loop this changes and the syntax is as below:
syntax:
// variable initialisation
while (condition) // condition check
{
/* statements to execute in loop */
// updating variable value
}
here is the sample program for while loop
// Write a program which prints first 5 multiples of 5 in reverse order
#include <stdio.h>
int main()
{
int i = 5;
while (i > 0)
{
printf("%d\n", i * 5);
i--;
}
}
output:
25
20
15
10
5
In both for loop and while loop, checks condition and executes the statement only if the condition is true, where are in do while statements are executed first and conditions are checked later.
do while
syntax:
do
{
/* statements to execute in loop */
// update value
} while (condition);
Prev << Conditional Statements in C
Next >> Pointers in C