Functions in c are sets of instructions grouped together to perform specific tasks. They help to avoid code repetition for similar tasks. By encapsulating a set of related instructions into a function, developers can better manage complexity and improve code readability.
main() is a function and the block of code written in main() is the body of main function.
int main()
{
// function body
}
In the same manner we can write any function as below:
int func_1()
{
// function body
}
The main() function is the entry point of a program, and from there, other functions can be invoked. But how other functions execute? this is possible by calling the function as shown below:
#include <stdio.h>
int func_1()
{
printf("in function func_1\n");
}
int main()
{
printf("in main function\n");
func_1();
printf("in main function\n");
}
output:
in main function
in function func_1
in main function
Explanation:
- We define a function called func_1.
- We define the main function, which serves as the entry point for the program.
- Inside main, we print a message indicating that we’re in the main function.
- We call the func_1 function, which prints a message indicating that we’re in func_1.
- We print another message in main to show that it resumes its task after executing func_1.
- Finally, we return 0 from main to indicate successful program execution.
In the same way we can use multiple functions as below:
#include <stdio.h>
int func_3()
{
printf("in func_3\n");
}
int func_2()
{
printf("in func_2\n");
func_3();
printf("in func_2\n");
}
int func_1()
{
printf("in function func_1\n");
}
int main()
{
printf("in main function\n");
func_1();
printf("in main function\n");
func_2();
printf("in main function\n");
}
output:
in main function
in function func_1
in main function
in function func_2
in function func_3
in function func_2
in main function
okay. But what is the need of functions in c? how it will help a programmer?
Uses of functions:
Modularization and Code Organization:
Functions allow for breaking down a large program into smaller, manageable, and reusable pieces. Each function can focus on a specific task, improving code organization and readability.
Code Reusability:
Developers can reuse functions in multiple parts of a program or even in different programs, saving time and effort by avoiding the need to write repetitive code for similar tasks.
Abstraction:
Functions can hide complex implementation details and provide a simplified interface. This abstraction allows other parts of the program to use the function without needing to understand how it works internally.
Terminologies of function in c
Function body:
The function body refers to the block of code within a function that contains the instructions and statements defining what the function does. It starts after the opening curly brace { following the function’s header (return type, function name, and parameters) and ends with the closing curly brace }. It contains the actual operations and logics of a function.
lets take an example of a function which adds two numbers:
int sum_of(int a, int b)
{
// function body
}
Arguments of a function:
Parameters (or arguments) are values passed to a function when it is called. The function uses these values to perform its operations.
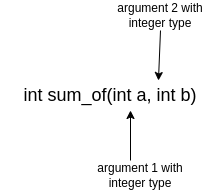
Function calling:
A function call is an expression that invokes a specific function, providing the necessary arguments. The function executes its code and may return a value.
int main()
{
// statements
sum_of(a,b); // calling sum_of function
// statements
}
Return type of a function:
The return type is the data type of the value that the function returns to the calling code. It’s specified before the function name in both the declaration and definition.

Function header:
The function header consists of the function name, return type, and the parameter list.
int sum_of(int a, int b) // function header
{
// function body
}
Function definition:
The function definition encompasses the complete specification of a function, including its header and body.
lets combine everything and write a program which adds two numbers given by user.
#include <stdio.h>
int sum_of(int a, int b)
{
/* function body */
int result;
result = a + b;
return result;
}
int main()
{
int a,b;
printf("enter a value:\n");
scanf("%d\n", &a);
printf("enter b value:\n");
scanf("%d\n", &b);
result = sum_of(a,b); // function calling
printf("The sum of %d and %d is %d\n", a, b, result);
}
Have you noticed that the function sum_of is defined above the main function, in which sum_of function is called? What if the function is defined below the main
function or below the caller?
When a function is defined above the caller, the compiler encounters the function definition before the calling of that function. The compiler is already aware of that function, and no issues arise. However, if the function is defined below and the compiler encounters the function call first, the compiler is not aware of what that function is about. So, the programmer has to inform the compiler about the function before calling it. This is possible by using a function declaration.
Function declaration:
A function declaration provides the function’s prototype, specifying its name, return type, and the types of its parameters (if any). It gives the compiler information about the function’s existence and signature.
int sum_of(int a, int b); // function declaration
Lets conclude the concept of functions by writing a simple program.
// Write a program which calculates x power y. if x = 5, y = 4 result = 625
#include <stdio.h>
int x_power_y(int a, int b); //function declaration
int main()
{
int x, y, result;
printf("Enter x value\n");
scanf("%d", &x);
printf("Enter y value\n");
scanf("%d", &y);
result = x_power_y(x, y); // function calling
if (result == -1)
{
printf("supports only for positive integers\n");
return 0;
}
printf("the value of %d power %d is %d\n", x, y, result);
return 0;
}
/* function definition */
int x_power_y(int a , int b) // function header, argument 1: int, argument 2: int
{
/* function body */
int r = a;
if (a < 0 || b < 0)
/* return error case */
return -1;
if (b == 0)
return 1;
while (--b > 0)
r *= a;
/* return value */
return r;
}
Argument passing
Functions in C accept arguments using two methods: Pass by value and Pass by reference.
Pass by Value:
In pass by value, the function receives a copy of the value of the argument. The function creates its own local variable and stores the copied value there. Changes made to the local variable within the function do not affect the original value.
sample program to understand pass by value:
#include <stdio.h>
int func1(int a)
{
printf("value of a =%d passed by main function\n", a);
a = 10;
printf("value of a = %d in func1() after modification\n", a);
}
int main()
{
int a = 100;
printf("value of a=%d in main function\n", a);
func1(a);
printf("value of a=%d in main after calling func1()\n", a);
}
output:
value of a=100 in main function
value of a =100 passed by main function
value of a = 10 in func1() after modification
value of a=100 in main after calling func1()
In the main
function, the value of the variable a
is set to 100
. When a
is passed to the function func1()
using pass-by-value, a copy of a
is made within the scope of func1()
.
Inside func1()
, the value of this copy is changed to 10
. However, because func1()
is working with a copy and not the original variable, this change does not affect the original a
in the main
function. As a result, when the program returns to main
, the value of a
remains 100
, which can be observed in the output.
Pass by Reference:
In pass by reference, the function receives the address (reference) of the argument. This allows the function to access and modify the actual variable using pointers. Any changes made to the variable within the function will directly affect the original variable in the calling code
Sample program to understand pass by reference
#include <stdio.h>
int func1(int *ptr)
{
printf("in func1(), value of a =%d passed by main() address=%p\n", *ptr, ptr);
*ptr = 10;
printf("value of a = %d in func1() after modification\n", *ptr);
}
int main()
{
int a = 100;
printf("value of a=%d in main(), address of a=%p\n", a, &a);
func1(&a);
printf("value of a=%d in main() after calling func1()\n", a);
}
output
value of a=100 in main(), address of a=0x7fffffffdca4
in func1(), value of a =100 passed by main() address=0x7fffffffdca4
value of a = 10 in func1() after modification
value of a=10 in main() after calling func1()
Inside func1()
, the value of a
is changed to 10
. Since func1()
is working with the reference to the original variable, this change directly modifies the original a
in the main
function. As a result, when the program returns to main
, the value of a
is now 10
, which can be observed in the output.
Prev << Endianess
Next >> Arrays in C