Datatypes in c – As the name implies, types of data. When writing code, you often encounter various kinds of data, such as whole numbers(-10, 0, 1000, 547384), decimal numbers(1.34, 8988.990), individual characters( ‘c’, ‘H’, ‘&’), and groups of characters(“onesandzeroverse”). To store this data in memory, you need to specify what type of data you’re dealing with. In languages like C, data is stored in variables and variables specify in which memory location the data is stored, for this variable a datatype needs to be declared indicating the kind of data that variable can hold.
So in simple words datatypes tell what data to be stored and also how much memory is required. Here are some common datatypes in C described in detail:
int (integer):
stores whole numbers positive, negative and zero.
Takes 4 bytes of memory
syntax:
int a = 10; // where a is a variable of int data type, assigned with value 10
As int data type typically occupies 4 bytes of memory, which is equivalent to 32 bits. It can store both negative and positive values. The range of values that can be accommodated in this type of variable is determined by these factors:
bit count:
With 32 bits available, there are 2^32 possible combinations.
sign bit:
In a signed int, the most significant bit (MSB) is used for sign representation. When the MSB is high (1), it indicates a negative value, and when it is low (0), it signifies a positive value.
Given these considerations, here’s the range of values for a signed int:
Minimum Negative Value: When the MSB is high (1), the minimum negative value is represented as -2^(31) which equals -2,147,483,648.
Maximum Positive Value: When the MSB is low (0), the maximum positive value is represented as 2^(31) – 1 which equals 2,147,483,647.
lets take few values and see how the values are stored:
int a = 50; here 50 is in decimal representation if we convert into binary we get 110010. This binary value can represent in 6 bits, but as it is mentioned as integer it stores in 32bit format as given below:
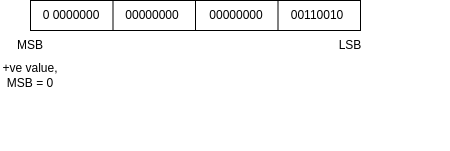
int a = -560; as it is a negative value, the process of storing in binary format is different from positive values.
- Binary format of 560 => 1000110000, as it is int data type in 32bit representation the binary value is, 00000000 00000000 000000010 00110000.
- Convert binary value into 2’s complement 11111111 11111111 11111101 11010000.
- 2’s complement of a number is stored if the given value is -ve.
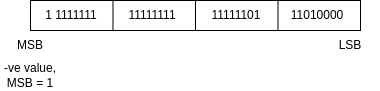
lets take an example of C code which stores integer value and prints the stored value:
#include <stdio.h>
int main()
{
/* store a value */
int a = 56;
/* print the value */
printf("The value of a is %d\n", a);
}
output:
The value of a is 56
%d in the printf statement specifies the datatype of the variable, every data type has its own format specifiers
char (character)
For storing characters
Takes 1 byte of memory
Syntax:
char c = ‘a’; // where c is a variable of char data type, assigned with value/character 'a'
As char data type in C is typically used to store a single character, such as a letter, digit, or symbol. It is represented using 8 bits (1 byte) of memory. Unlike the int data type, char is not inherently signed or unsigned.
Here’s how the char data type works in terms of its bit representation:
bit count:
char uses 8 bits, which gives it a total of 2^8 (256) possible combinations.
sign bit:
Unlike int, char does not have a separate sign bit. It uses all 8 bits to represent the character’s value.
Given these considerations:
The range of values for a char data type is typically from 0 to 255.
The characters which are assigned correspond to a specific ASCII value. Each character has its own ASCII value. as given in the example int c = ‘a’; the ASCII value of ‘a’ is 97, so when you store ‘a’ in a char variable, the actual value stored is 97 in binary format 01100001
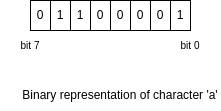
The ASCII values of alphabets fall in the range of 65 to 90 for capital letters(A to Z) and 97 to 122 for small letters(a to z).
Lets take an example of C code which stores a character value:
#include <stdio.h>
int main()
{
/* store a character */
char c = 'e';
/* print the character */
printf("The value of c is %c\n", c);
output:
The value of c is e
float
Float data types store real numbers like 3.4, 2345.66, 78.000, -867.4, etc. The size of a float data type is 4 bytes.
For example:
float f = 54.35;
However, unlike integers, floats don’t store their values through direct binary conversion. Instead, they follow specific rules. The 4 bytes of a float are divided into three parts:
Sign Bit: The sign bit determines whether the value is positive (0) or negative (1).
Exponent Bits: The exponent is represented by 8 bits and indicates the binary scientific notation’s exponent value. For float number of exponent bits are 8.
Mantissa Bits: The mantissa, or significand, represents the value before and after the decimal point. for float number of exponent bits are 23.
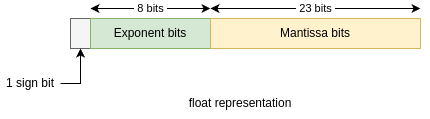
Steps to convert floating value to its binary representation
Lets take 54.35 as an example float value.
1. Find whether float value is positive or negative value.
sign bit is 0 for positive values, 1 for negative values.
54.35 is a positive value, so sign bit is 0.
2. Convert the real value to its binary value.
The binary representation of 54.35 is 110110.11
3. Represent the binary value in scientific notation.
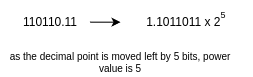
4. Calculate exponent value.
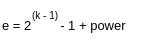
k, the number of exponent bits
power, power value in scientific binary notation
from step 3, power = 5, for float k = 8 the value of exponent is
e = 127 + 5
e = 132
binary representation of e is 10000100, this is the exponential bits.
5. Get mantissa bits
The bits after decimal point in scientific notation represents mantissa bits.
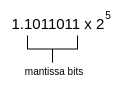
so the mantissa bits are 10110110000000000000000 as it have to represent in 23 bits
Now we got all the parts of floating value binary, the final binary representation is:

Data types available in C
In C programming, the size of data types can vary depending on the system and compiler being used. Here’s a list of common datatypes in C for a 64-bit system, along with their typical sizes and format specifiers for use with printf
:
datatype | size in bytes | format specifier |
int | 4 | %d |
char | 1 | %c |
float | 4 | %f |
unsigned int | 4 | %u |
short int | 2 | %hd |
unsigned short int | 2 | %hu |
long int | 8 | %ld |
unsigned long int | 8 | %lu |
long long int | 8 | %lld |
unsigned long long int | 8 | %llu |
double | 8 | %lf |
long double | 16 | %Lf |
void | N/A | N/A |
Prev << Basics of C
Next >> Operators in C