Endianness refers to the order in which bytes are stored in computer memory for multibyte data types like integers and floating-point numbers. There are two common types of endianness: little-endian and big-endian.
Little-Endian:
In a little-endian system, the least significant byte is stored first (at the lowest memory address), followed by the more significant bytes. This is analogous to how we write numbers in decimal, starting from the rightmost digit (least significant) to the left (most significant). Little-endian is the most prevalent endianness for modern computer architectures, including x86 and x86-64.
lets take an integer value of 0x12345678. (taking value in hexadecimal to make explanation simpler.
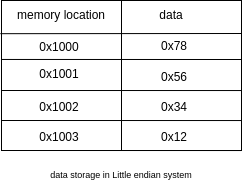
Big-Endian:
In a big-endian system, the most significant byte is stored first (at the lowest memory address), followed by the less significant bytes. This is similar to how we write numbers in English, with the most significant digit (the leftmost one) coming first. Big-endian is common in network protocols and older computer architectures.
The choice of endianness has implications for data interchange between systems with different endianness, such as in networking or reading binary files. When transferring multibyte data between systems with different endianness, the bytes need to be rearranged to ensure correct interpretation of the data.
the same value 0x12345678 is stored in big endian system as follows:
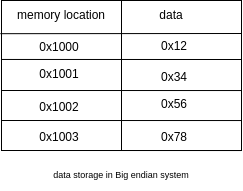
How to find Endianess of a system?
We know that an integer variable typically occupies 4 bytes of memory. For example, at memory addresses 0x100, 0x101, 0x102, and 0x103). When a value is assigned to an integer variable, the value is stored in memory according to the system’s endianness.
Let’s assume we assign the value 1 to this integer variable. Since 1 is the least significant byte (LSB), its storage location will depend on whether the system is little-endian or big-endian:
Little-endian system:
The LSB is stored in the lowest memory address, so the value 1 will be stored at memory address 0x100.
Big-endian system:
The LSB is stored in the highest memory address, so the value 1 will be stored at memory address 0x103.
But how can we determine which endianness is used by the system?
This is where pointers can be helpful. A character pointer points to a single byte of memory. If we assign the address of the integer variable to a character pointer, the pointer will hold the starting address of the integer variable, regardless of the system’s endianness. In this case, the pointer will point to address 0x100.
Now, we can check the value at this memory address by dereferencing the pointer:
If the value at address 0x100 is 1, then the system is little-endian.
If the value at address 0x100 is 0, then the system is big-endian.
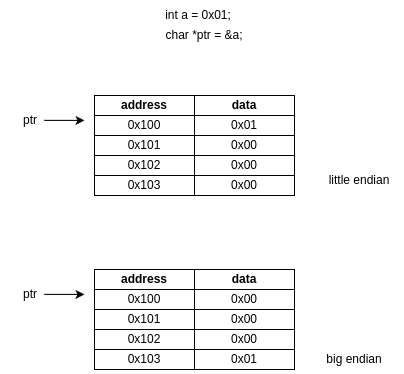
Program to find the whether the sytem is little endian or big endian
#include <stdio.h>
int main()
{
int a = 0x01;
char *ptr = (char *)&a;
if (*ptr == 0x01)
printf("the system is little endian\n");
else
printf("The system is big endian\n");
}
Prev << Pointers in C
Next >> Functions in C