Operators in C are keywords or special symbols that are used to perform operations on operands. Operands are the values or variables on which the operations are performed. C provides a wide variety of operators that can be categorized into the following types:
1. Arithmetic Operators:
Arithmetic operators in C are used for mathematical calculations and follow the same principles as basic arithmetic taught in primary education. These operators operate on values or variables and include:
- +(addition)
- -(subtraction)
- *(multiplication)
- / (division)
- % (modulo or remainder)
here is the program to add two numbers:
#include <stdio.h>
int main()
{
int a = 10, b = 20, c;
/* add a and b */
c = a + b;
printf("The sum of a and b = %d\n", c);
}
output:
The sum of a and b = 30
2. Relational Operators:
Relational operators in C are used to compare two values or variables, They determine how one value compares to another and return a true or false result based on the comparison.
- == (equal to)
- != (not equal to)
- < (less than)
- > (greater than)
- <= (less than or equal to)
- >= (greater than or equal to)
These operators are crucial for making decisions and controlling the flow of a program based on comparisons between different variables or values. If the expression result is true, its value is 1. If the expression result is false, its value is 0.
for example:
#include <stdio.h>
int main()
{
int a;
int b = 10, c = 5;
a = b > c;
printf("a=%d\n", a);
}
in the above program b is greater than 5 so the expression b > c is true, a is assigned with 1.
a=1
Sample programs using these operators are discussed in conditional statements chapter.
3. Logical Operators:
These operators in C are used to perform logical operations and include the following:
&& (Logical AND)
Below table shows the output of logical AND operator with given two values:
input 1 (X) | intput 2 (Y) | Z = X && Y |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
|| (logical OR)
Below table shows the output of logical OR operator with given two values:
intput 1 (X) | input 2 (Y) | Z = X || Y |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
! (logical NOT)
Below table shows the output of logical NOT operator with given value:
input (X) | Z = !X |
---|---|
0 | 1 |
1 | 0 |
Sample program to understand relational and logical operatiors.
Find a given number is in between 40 and 70.
input: 39
output: the number is outside 40 and 70
#include <stdio.h>
int main()
{
int num;
printf("Enter a number:");
scanf("%d", &num);
if ((num > 40) && (num < 70))
printf("The number is inside 40 and 70\n");
else
printf("The number is outside 40 and 70\n");
}
input:
57
output:
The number is inside 40 and 70
4. Bitwise Operators:
All the other operators deal with values but Bitwise operators in C are used to perform operations at the bit level and include the following:
& (bitwise AND)
The bitwise AND (&) operation is a binary operation that operates on each bit of two integers independently, comparing corresponding bits and producing a result where a bit is set (1) only if the corresponding bits in both operands are set (1).
In a logical AND 1 && 5, both are high, result is true or 1 but in bitwise AND 1 & 5, binary value of 1 in 8 bit representation is 0000 0001, binary value of 5 is 0000 0101. bit wise AND of these two is as follows:
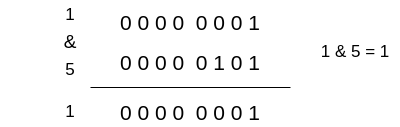
when both bit of a particular bit number is high, the resultant bit value is also high
4 && 0, one is low, result is low or 0, 4 & 0 also gives 0
lets take a bigger number 456 & 322
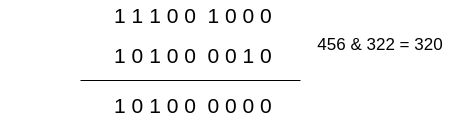
as explained above all bit wise operations work similar
| (bitwise OR)
The bitwise OR (|) is a binary operation that operates on each bit of two integers independently, comparing corresponding bits and producing a result where a bit is set (1) if at least one of the corresponding bits in the operands is set (1).

^ (bitwise XOR)
The XOR (exclusive OR) operation is a binary operation that takes two binary digits (bits) as operands and outputs 1 if the number of ones in the operands is odd, and 0 if the number of ones is even. The XOR operation is commonly denoted by the symbol ‘^’.

~ (bitwise NOT)
The bitwise NOT operation (also known as bitwise negation) is a unary operation that flips all the bits of a binary number, changing 0s to 1s and 1s to 0s. It’s represented by the symbol ‘~’.
if A is 10101010, then ∼A (bitwise NOT of A) is 01010101.
<< (left shift)
The left shift (<<) operator is a bitwise operator in C that shifts the bits of a binary number to the left by a specified number of positions.
Syntax: operand << number_of_positions
operand: The value you want to shift.
number_of_positions: The number of positions to shift the bits to the left.
lets take few examples to understand left shift
5 << 2, binary value of 5 is 0000 0101

15 << 4, binary value of 15 is 00000 1111

as seen from the above examples, instead of converting operand into binary form, shifting them by number_of_positions, and converting the new binary to decimal. There is a shortcut for this as given below:
result = operand * 2^number_of_positions
5 << 2, 5 * 2^2 gives 20 and 15 << 4, 15 * 2^4 gives 240.
>> (right shift)
The right shift (>>) operator is a bitwise operator in C that shifts the bits of a binary number to the right by a specified number of positions.
Syntax: operand >> number_of_positions
operand: The value you want to shift.
number_of_positions: The number of positions to shift the bits to the right.
lets take few examples to understand right shift
8 >> 2, binary value of 8 is 0000 1000

The bitwise operators are commonly used in low-level programming, embedded systems, and bit manipulation tasks.
5. Assignment Operators
Assignment operators are used to assign values to variables. They combine the assignment operation with arithmetic or bitwise operations. These operators are a shorthand way to modify the value of a variable based on its current value.
Here’s a list of common assignment operators in C:
Assignment Operator (=):
Assigns the value on the right to the variable on the left.
int x = 10; // Assigns the value 10 to variable x
Addition and Assignment Operator (+=):
Adds the value on the right to the variable and assigns the result to the variable.
int x = 5;
x += 3; // Equivalent to: x = x + 3 (x is now 8)
Bitwise AND and Assignment Operator (&=):
Performs a bitwise AND between the variable and the value on the right and assigns the result to the variable.
int x = 5; // Binary: 0101
x &= 3; // Equivalent to: x = x & 3 (x is now 1, Binary: 0001)
The list of other available assignment operators:
-= (subtraction and assignment)
*= (multiplication and assignment)
/= (division and assignment)
%= (modulo and assignment)
|= (bitwise OR and assignment)
^= (bitwise XOR and assignment)
<<= (left shift and assignment)
>>= (right shift and assignment)
These assignment operators provide a compact way to modify variables based on their current values.
(++)Increment and (−−)Decrement
The increment (++) and decrement (−−) operators are used to increase or decrease the value of a variable by 1, respectively. These operators can be applied to variables of numeric types (integers, floats, etc.).
Here’s a detailed explanation of the increment and decrement operators:
Increment Operator (++):
Syntax: ++variable or variable++
Usage:
++variable increments the value of variable by 1 and then uses the new value.
variable++ uses the current value of variable and then increments it by 1.
int x = 5;
int y = ++x; // Increment x by 1, then assign it to y (x becomes 6, y is 6)
int x = 5;
int y = x++; // assign it to y and Increment x by 1, (x becomes 6, y is 5)
Decrement Operator (−−):
Syntax: −−variable or variable−−
Usage:
−−variable decrements the value of variable by 1 and then uses the new value.
variable−− uses the current value of variable and then decrements it by 1.
int x = 10;
int y = --x; // decrement x by 1, and assign 9 to y (x becomes 9, y is 10)
int x = 10;
int y = x--; // assign x to y and then decrement it by 1 (x becomes 9, y is 10)
6. Comma operator (,)
The comma operator lets you evaluate multiple expressions within a single statement and is often used to sequence multiple expressions.
Here’s the syntax and usage of the comma operator:
expression1, expression2;
It is commonly used in variable initialisation, (loop statements and functions which will be disccussed later)
int a = 5, b = 10; // Multiple variables can be initialized in a single statement using the comma operator
7. Conditional operator (?:)
The conditional operator, also known as the ternary operator, is unique in C as it takes three operands. It evaluates a condition and selects one of two values based on the result. It has the following syntax:
condition ? statement_if_true : statement_if_false
The expression evaluates as follows:
- If the
condition
is true, thestatement_if_true
is executed. - If the
condition
is false, thestatement_if_false
is executed.
Here’s an example to illustrate the usage of the conditional operator:
#include <stdio.h>
int main() {
int a = 10;
int b = 5;
int max = (a > b) ? a : b;
printf("The maximum value is: %d\n", max); // Output: The maximum value is: 10
return 0;
}
In this example, the expression (a > b) ? a : b
evaluates whether a
is greater than b
. If it is true, it assigns the value of a
to max
; otherwise, it assigns the value of b
.
sizeof operator is discussed in datatypes chapter
*(dereference), . (access member of a structure), -> (access member of a structure through a pointer), & (address of) operators is discussed in the pointers chapter.
Precedence and Associativity
Precedence and associativity determine the evaluation of an expression when it contains more than one operator.
Consider the example expression: a = 2 * 10 + 50 / 2;.
There are four operators in this expression, and the final result can vary depending on the order of evaluation. For instance, if the order of evaluation is *, +, /, and =, the result will be 35. However, if the order is /, +, *, and =, the result will be 70.
To avoid such confusion, C provides a set of rules to evaluate expressions consistently.
Precedence levels act as rankings for operators, with higher-ranked operators being executed first.
Associativity dictates the order of evaluation when multiple operators with the same precedence level are present.
Precedence and associativity table of operators:
Operator | Description | Precedence level | Associativity |
( ) [ ] -> . | Function call Array subscript Arrow operator Dot operator | 1 | Left to Right |
+ – ++ — ! ~ * & (data type) sizeof | Unary plus Unary minus Increment Decrement Logical NOT One’s complement Indirection Address Type cast Size in bytes | 2 | Right to Left |
* / % | Multiplication Division Modulus | 3 | Left to Right |
+ – | Addition Substraction | 4 | Left to Right |
<< >> | Left shit Right shit | 5 | Left to Right |
< <= > >= | Less than Less than or equal to Greater than Greater than or equal to | 6 | Left to Right |
== != | Equal to Not equal to | 7 | Left to Right |
& | Bitwise AND | 8 | Left to Right |
^ | Bitwise XOR | 9 | Left to Right |
| | Bitwise OR | 10 | Left to Right |
&& | Logical AND | 11 | Left to Right |
|| | Logical OR | 12 | Left to Right |
? : | Conditional operator | 13 | Right to Left |
= *= /= %= += -= &= ^= |= <<= >>= | Assignment operators | 14 | Right to Left |
, | Comma operator | 15 | Left to Right |
Sample expressions:
- a = 2 * 10 + 50 / 2;
- In the above expression, * and / have the highest precedence levels compared to the other operators. Since * and / share the same precedence level, the expression evaluates based on left-to-right associativity. The evaluation of the expression proceeds as follows:
- a = 20 + 50 / 2;
- a = 20 + 25;
- a = 45;
- a = 5 + 16 / 2 * 4 – 3;
- The expression evaluates as follows:
- a = 5 + 8 * 4 – 3
- a = 5 + 32 – 3
- a = 37 – 3
- a = 34
- if a = 10, b = 25, c = 43;
x = a <= b || b == c;- x = 1 || b == c;
- x = 1 || 0
- x = 1
- if a = 10, b = 23, c = 4, d = 20;
z = a++ + ++b * c– – –d;- unary operators have the higher precedence level compared to mathematical operators
- z = 10 + 24 * 4 – 19;
- z = 87;
- y = ++x++;
- The expression y = ++x++; is invalid because it tries to apply both the pre-increment (++x) and post-increment (x++) operators to x simultaneously, which leads to undefined behaviour.
- x = y = z = 5;
- all x, y and z are assigned with 5;
- if x = 10, y = 0, z = 2;
x += y = z *= 5;- x += y = 10;
- x += 10;
- x = 20;
- if x = 8, y = 2;
a = x >> 1 * y;- a = 8 >> 1 * 2;
- a = 8 >> 2;
- a = 2;
- if a = 2, b = 5, c = 7, d = 1, e = 10, f = 15;
z = a + b – (c + d) * 3 % e + f / 9;- z = 10
- Inorder to avoid confusion and improve readability, expressions can maintain brackets as
x = ((22 – 4) / (3 + 3)) * 4- brackets are evaluated first
- x = (18 / 6) * 4
- x = 3 * 4
- x = 12;
Prev << Datatypes in C
Next >> Type casting