As we have seen in the chapter on arrays, arrays are collections of elements of the same type. A 2-dimensional (2-D) array is essentially an array of arrays, meaning it is a collection of 1-dimensional (1-D) arrays of the same type.
For example, if you have a collection of 10 integer elements grouped together, it forms a 1-D array. If you then group several such 1-D arrays together, you create a 2-D array.
Rows and Columns
To access an element in a 2-D array, you first need to identify the 1-D array (or row) that contains the element. The row index specifies which 1-D array within the 2-D array you are dealing with. Once the row is determined, the column index is used to locate the specific element within that 1-D array.
Row Index: Identifies the 1-D array within the 2-D array.
Column Index: Identifies the specific element within the selected 1-D array.
Representation of 2-D arrays in C
data_type arr[rows][columns];
data_type: datatype of elements in the array
rows: number of 1-D arrays
columns: maximum number of elements that a 1-D array can contain.
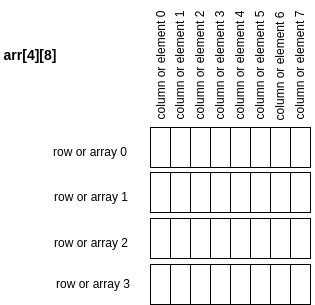
Total number of elements in a 2-D array
The total number of elements in a 2-D array = rows x columns
all the elements are same data type
Examples:
int arr[4][5];
The array represents integer type of data with 4 1-D arrays each contain 5 integer elements.
char arr[5][6];
The array represents 5 1-D arrays, each arrays contains 6 character type of elements.
Initialisation of 2-D array
1. Similar to 1-D array, 2-D arrays are also initialised with braces, but inner braces are added to represent each 1-D array as shown below:
int arr[3][6] = {{1, 46, 34, 78, 88, 23, 101}, {45, 89, 90, 22, 67, 11}, {89, 45, 66, 77, 89, 90}};
2. At the time of initialisation, number of elements in a 1-D array can be less than the number of columns as shown below
int arr[3][6] = {{1, 46, 34}, {89, 90, 22}, {89, 45, 66, 77, 89, 90}};
// the above initialisation is considered as shown below
int arr[3][6] = {{1, 46, 34, 0, 0, 0}, {89, 90, 22, 0, 0, 0}, {89, 45, 66, 77, 89, 90}};
3. The inner braces may not be specified while initialisation, elements are separated by the compiler based on the number of columns. as shown below
int arr[3][6] = {1, 46, 34, 78, 88, 23, 101, 45, 89, 90, 22, 67, 11, 89, 45, 66};
// the above initialisation is taken as shown below
int arr[3][6] = {{1, 46, 34, 78, 88, 23}, {101, 45, 89, 90, 22, 67}, {11, 89, 45, 66, 0, 0}};
4. To have a good visual understanding, 2-D arrays can also initialised as below:
char arr_ch[4][6] = {
{'a', 'b', 'A', 'Z', 'e', '1'},
{'z', 'f', 'q', 'r', 'o', 'x'},
{'d', '1', '0', 'v', '3', 'l'},
{'v', 'f', '2', 'Z', '/', 'G'},
{'a', 'O', 'A', '9', 'S', 'b'},
{'a', '2', 'a', 'j', 'w', 'k'},
};
5. Its optional to specify the first dimension while the initializing but the second dimension should always be present for example:
int arr[][5] = {{1, 5, 3, 8, 9}, {3, 45, 6, 4, 6}, {5, 6, ,4 ,55, 9}};
Accessing elements in 2-D array
To access an element in a 2-D array, you need to specify both the row and column indices, as shown in the example below:
int arr[1][4];
The value of the element located at row 1 and column 4 in the 2-D array arr
is assigned to the variable a
.
Sample program to display elements in 2-D array
#include <stdio.h>
int main()
{
int arr[3][6] = {
{23, 44, 66, 12, 1},
{101, 15, 16, 98},
{77}
};
int i, j;
/* traverse through rows */
for (i = 0; i < 3; i++) {
printf("row %d elements are: ", i);
/* traverse through columns */
for (j = 0; j < 6; j++) {
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
output:
row 0 elements are: 23 44 66 12 1 0
row 1 elements are: 101 15 16 98 0 0
row 2 elements are: 77 0 0 0 0 0
2-D array from stdin
Sample program to understand how user provided input data is assigned to 2-D array.
#include <stdio.h>
int main()
{
int i,j;
int arr[3][6];
for (i = 0; i < 3; i++) {
for (j = 0; j < 6; j++) {
printf("enter arr[%d][%d]=", i, j);
scanf("%d", &arr[i][j]);
}
}
/* traverse through rows */
for (i = 0; i < 3; i++) {
/* traverse through columns */
printf("row %d elements are: ", i);
for (j = 0; j < 6; j++) {
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
input:
enter arr[0][0]=4
enter arr[0][1]=5
enter arr[0][2]=3
enter arr[0][3]=7
enter arr[0][4]=99
enter arr[0][5]=45
enter arr[1][0]=34
enter arr[1][1]=67
enter arr[1][2]=12
enter arr[1][3]=90
enter arr[1][4]=67
enter arr[1][5]=44
enter arr[2][0]=34
enter arr[2][1]=200
enter arr[2][2]=78
enter arr[2][3]=45
enter arr[2][4]=213
enter arr[2][5]=90
output:
row 0 elements are: 4 5 3 7 99 45
row 1 elements are: 34 67 12 90 67 44
row 2 elements are: 34 200 78 45 213 90
Program to Add two matrices
#include <stdio.h>
int main()
{
int i,j;
int a[3][2];
int b[3][2];
for (i = 0; i < 3; i++) {
for (j = 0; j < 2; j++) {
printf("enter a[%d][%d] ", i, j);
scanf("%d", &a[i][j]);
}
}
for (i = 0; i < 3; i++) {
for (j = 0; j < 2; j++) {
printf("enter b[%d][%d] ", i, j);
scanf("%d", &b[i][j]);
}
}
printf("sum of matrix a and b is \n");
/* traverse through rows */
for (i = 0; i < 3; i++) {
/* traverse through columns */
for (j = 0; j < 2; j++) {
printf("%d ", a[i][j] + b[i][j]);
}
printf("\n");
}
}
input
enter a[0][0] 1
enter a[0][1] 5
enter a[1][0] 3
enter a[1][1] 7
enter a[2][0] 3
enter a[2][1] 8
enter b[0][0] 0
enter b[0][1] 3
enter b[1][0] 5
enter b[1][1] 6
enter b[2][0] 7
enter b[2][1] 8
output
sum of matrix a and b is
1 8
8 13
10 16